Standard integration via SDK - MF Holdings Import
Build a holistic view of a userβs mutual fund investments, with standard integration (where SDK provides the readymade UI) πππ
With smallcase Gateway, you can easily import the latest snapshot and transaction details of a userβs mutual fund holdings natively in a seamless manner
This guide contains:
- Different ways to integrate and import MF holdings
- Holdings data structure
- Sample holdings data
- Implementation details for standard integration (SDK + API)
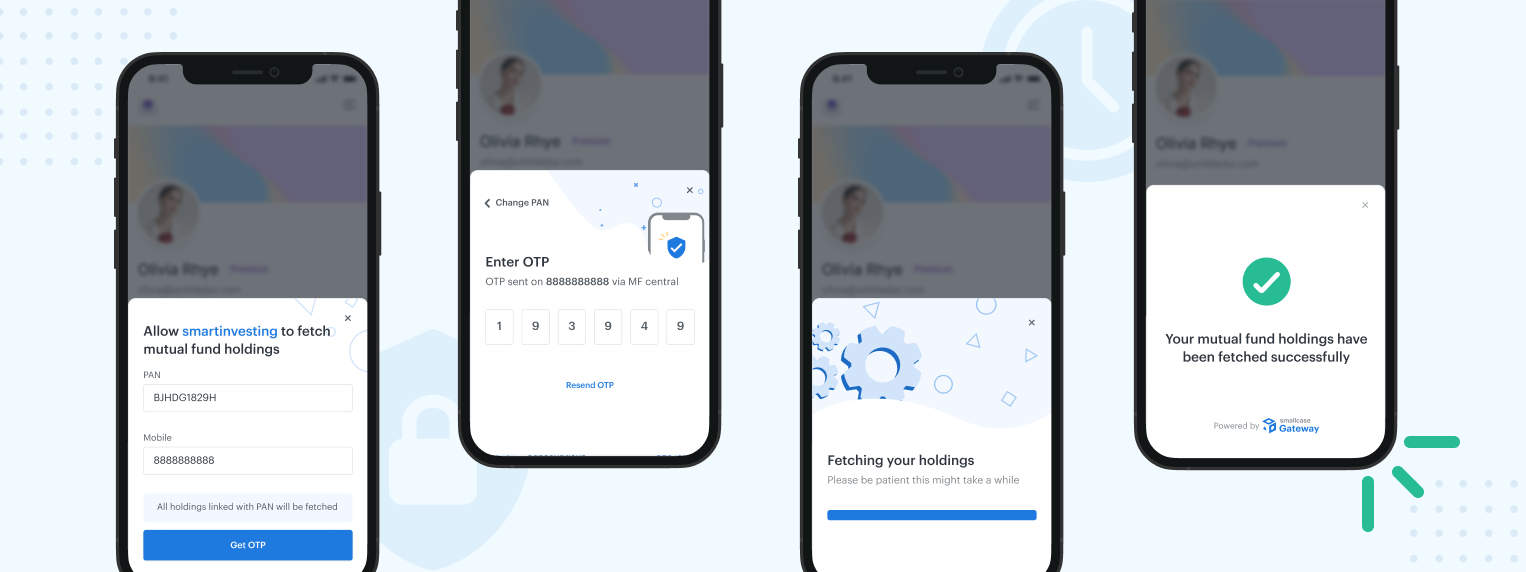
The user journey
Different ways to integrate
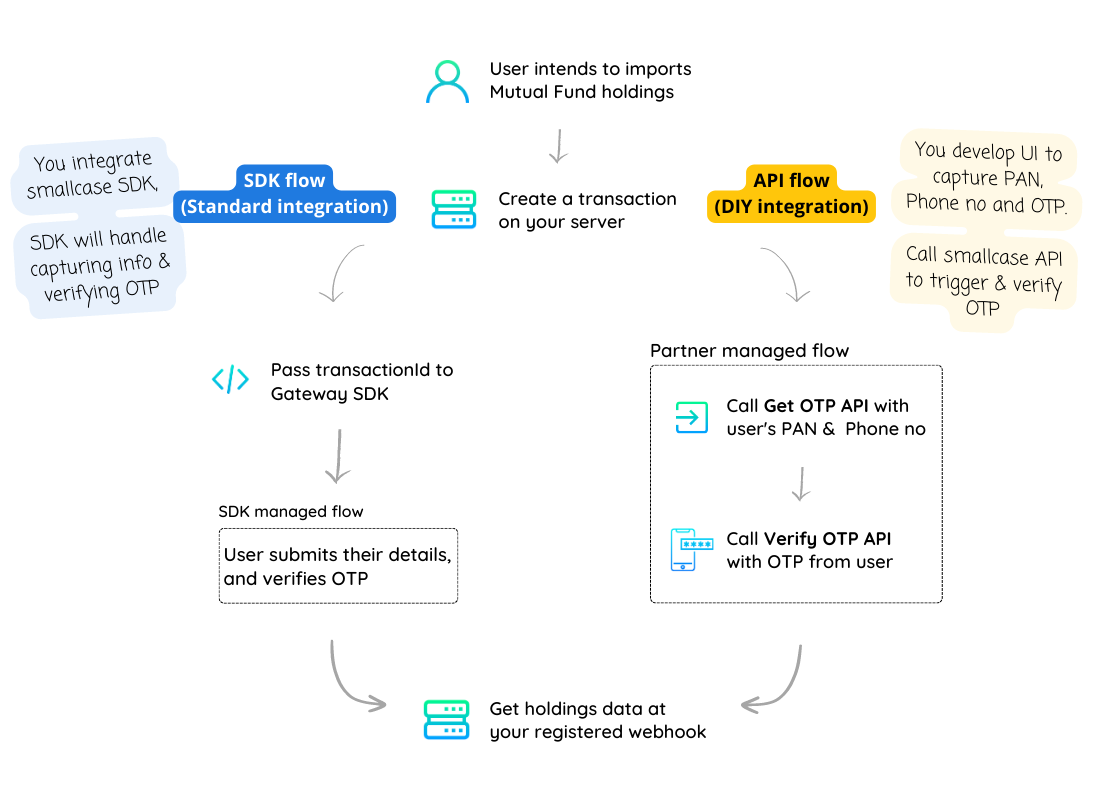
Implemenation sequence diagram
This diagram suggests the sequence of interactions between different entities involved:
- Your frontend application
- Gateway SDK residing in your frontend app
- Your server
- Gateway API
Response structure
field | type | description |
---|---|---|
summary.schemeDetails | SchemeDetails | Array of scheme details |
summary.portfolio | Portfolio | Portfolio Summary |
summary.exited | Includes those folios, which have currentValue and units as zero "data": { "0-0": "summary | |
transactions | Transactions | Array of all the transactions |
userInfo | UserInfo | User information |
fromDate | string | The date from which the transaction data was requested |
toDate | string | The date up till which the transaction data was requested (this will always be the present date i.e. the date on which the Mutual fund holdings were imported) |
snapshotDate | string | The date represents the snapshot time, when the latest holdings were fetched (this will always be the present date i.e. the date on which the Mutual fund holdings were imported |
transactionId | string | The transactionId with which the holdings were imported |
gateway | string | Gateway name |
checksum | string | Checksum for the webhook response |
timestamp | string | Timestamp when the webhooks were sent |
Object.summary.schemeDetails
field | type | description |
---|---|---|
isin | string | a 12-digit alphanumeric code that uniquely identifies a specific security |
folios | array | Array of folios |
scheme | string | Scheme name |
schemeCode | string | Scheme code |
amc | string | Amc name |
amcCode | string | Amc code |
assetType | string | Asset type |
currentValue | number | Current value of the scheme |
exitLoad | string | Exit load remarks |
entryLoad | string | Entry load remarks |
units | number | Number of units for the scheme |
nav | number | Net asset value of the scheme |
analytics | Analytics | Analytics object |
fundType | string | Type of fund |
exitLoadRemarks | string | Exit load remarks |
Object.summary.schemeDetails.folios
field | type | description |
---|---|---|
folioNumber | string | Folio number of the scheme |
brokerName | string | Name of the broker |
brokerCode | string | Broker code |
units | number | Number of units associated with the folio |
isDemat | boolean | |
investedValue | number | Invested amount in the scheme |
currentValue | number | Current Value |
currentReturns | number | Current returns |
totalReturns | number | Total returns |
realisedReturns | number | Realised returns |
averagePrice | number | AveragePrice of the scheme |
currentReturnsPercentage | number | Current returns percentage |
xirr | number | Folio level xirr |
rtaCode | string | |
kycStatus | string | KYC verified status 3 - Verified |
string | ||
decimalUnits | number | Decimal Units- Up to 3 decimal Points |
decimalAmount | number | Decimal Units for Amount β Upto 2 decimal Points |
decimalNav | number | Decimal Units for NAV β upto 4 decimal Points |
lastTrxnDate | string | Last Transaction Date |
openingBal | number | Opening Balance of the holdings |
closingBal | string | closing Balance of the holdings |
lastNavDate | string | Last NAV Date β which is available for the scheme |
bank | Bank | bank details |
transactionFlags | TransactionFlags | |
lienUnitsFlag | string | |
availableUnits | number | |
lockInUnits | number | For funds with lock-in perioud, lockInUnits is calculated from transaction history |
availableAmount | number | |
mobile | string | |
taxStatus | string |
Object.summary.schemeDetails.folios.bank
field | type | description |
---|---|---|
accountType | string | account type |
name | string | bank name |
branch | string | branch name |
city | string | city |
pincode | string | pin code |
micr | string | micr code |
ifsc | string | ifsc code |
neftifsc | string | neftifsc code |
Object.summary.schemeDetails.folios.transactionFlags
field | type | description |
---|---|---|
purAllow | string | |
redAllow | string | |
swtAllow | string | |
sipAllow | string | |
stpAllow | string | |
swpAllow | string |
Object.summary.schemeDetails.analytics
field | type | description |
---|---|---|
timestamp | string | Timestamp of the response |
xirr | number | Scheme level xirr |
investedValue | number | Invested amount in the scheme |
currentReturns | number | Current returns |
averagePrice | number | AveragePrice of the scheme |
currentReturnsPercentage | number | Current returns percentage |
schemeWeight | number | Scheme weight is the ratio of the scheme w.r.t. the current value of the portfolio. |
realisedReturns | number | Realised returns |
totalReturns | number | Total returns |
sectorAllocationPercentage | array | Sector allocation percentage |
Object.summary.schemeDetails.analytics.sectorAllocationPercentage
field | type | description |
---|---|---|
sectorName | string | Sector name |
value | number | Sector value |
Object.summary.portfolio
field | type | description |
---|---|---|
xirr | number | Portfolio level xirr |
schemeCount | number | Total scheme count |
currentReturns | number | Current returns |
realisedReturns | number | Realised returns |
totalReturns | number | Total returns |
sectorAllocationPercentage | array | Sector allocation percentage |
Object.summary.portfolio.sectorAllocationPercentage
field | type | description |
---|---|---|
sectorName | string | Sector name |
value | number | sector value |
Object.transactions
field | type | description |
---|---|---|
isin | string | a 12-digit alphanumeric code that uniquely identifies a specific security |
folioNumber | string | Folio number |
amc | string | Amc name |
amcCode | string | Amc code |
schemeCode | string | Scheme code |
scheme | string | Scheme name |
transactionDate | string | Transaction date |
postedDate | string | Date at which transaction was posted |
trxnDescription | string | Transaction Description β Possible values β Purchase, Redemption, Switch, SIP & NFTs description |
trxnUnits | number | Transaction amount |
purchasePrice | number | NAV β at which the Transaction was executed |
stampDuty | number | Stamp Duty applied charges |
totalTax | number | Total tax deducted |
transactionMode | string | Transaction Mode Possible Values - "N - Normal ", M - missed , R - Reversal |
cashFlow | string | Possible Values - "SELL" , "DEPOSIT" |
checkDigit | number | |
string | email id | |
trxnCharge | number | |
sttTax | number | |
tax | number | |
analytics | Analytics | Analytics object |
Object.transaction.analytics
field | type | description |
---|---|---|
transactionType | string | Type of transaction -DEPOSIT , WITHDRAW , REJECTED DEPOSIT , NA |
transactionStatus | string | Status of the transaction -SUCCESS , ADJUSTMENT , VOID |
transactionDescription | string | Transaction description -PURCHASE , REDEMPTION , SIP , SWP , DIVIDEND , SWITCH IN , SWITCH OUT |
exited | boolean | Denotes whether the transaction belongs to an exited folio or not If exited=true, analytics will not be present |
Object.userInfo
field | type | description |
---|---|---|
pan | string | PAN of the user |
name | string | Name of the user |
phone | string | Phone number of the user |
string | Email of the user | |
address | object | Address information of the user |
emails | array | array of emails (string) |
kycStatus | string | KYC verified status 3 - Verified |
taxStatus | string | |
bankAccounts | array of Bank | bank accounts |
dpIds | array | array of dpIds (string) |
Sample response
{
"fromDate": "1-Jan-1986",
"toDate": "7-Dec-2022 ",
"snapshotDate": "2022-12-07T12:21:04.934Z",
"transactionId": "TRX_cd0ca32353b14f6b92bcd0dfb0b05c32",
"gateway": "gatewaydemo",
"timestamp": "2025-01-01T12:00:00.000Z",
"checksum": "a604cc4e0a4b99a027cd1782c2551e33aab3fbc6a712797c29b8c4b555f9e529",
"summary": {
"schemeDetails": [
{
"isin": "INF200K01RJ1",
"folios": [
{
"folioNumber": "xxxxxxxx",
"brokerName": "INZ000208032",
"brokerCode": "INZ000208032",
"isDemat": "N",
"units": 184.47399999999996,
"investedValue": 40498.228052800005,
"currentValue": 47255.321025,
"currentReturns": 6757.092972199993,
"totalReturns": 6757.092972199993,
"realisedReturns": 0,
"averagePrice": 219.53352804622875,
"currentReturnsPercentage": 16.684910172836105,
"remarks": "live data unavailable",
"xirr": 11.577337973980805,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 47255.321025,
"lastNavDate": "12-DEC-2022",
"bank": {
"accountNo": null,
"accountType": null,
"name": null,
"branch": null,
"city": null,
"pincode": null,
"micr": null,
"ifsc": null,
"neftifsc": null
},
"transactionFlags": {
"purAllow": null,
"redAllow": null,
"swtAllow": null,
"sipAllow": null,
"stpAllow": null,
"swpAllow": null
},
"lienUnitsFlag": null,
"lockInUnits": 0,
"availableUnits": null,
"availableAmount": null,
"mobile": null,
"taxStatus": null
}
],
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"schemeCode": "D81G",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 184.47399999999996,
"nav": 256.1625,
"currentValue": 47255.321025,
"fundType": "NA",
"exitLoadRemarks": "NA",
"analytics": {
"investedValue": 40498.228052800005,
"averagePrice": 219.5335280462288,
"timestamp": "2023-02-02T12:24:56.413Z",
"realisedReturns": 0,
"currentReturns": 6757.092972199993,
"totalReturns": 6757.092972199993,
"currentReturnsPercentage": 16.684910172836105,
"sectorAllocationPercentage": [],
"remarks": "live data unavailable",
"schemeWeight": 62.37944023707425,
"xirr": 11.577337973980805
}
},
{
"isin": "INF846K01DP8",
"folios": [
{
"folioNumber": "abc12345",
"brokerName": "DIRECT",
"brokerCode": "INZ000208032",
"isDemat": "N",
"units": 575.2789664917238,
"investedValue": 25998.528979999995,
"currentValue": 28499.32,
"currentReturns": 2500.791020000004,
"totalReturns": 2500.791020000004,
"realisedReturns": 0,
"averagePrice": 45.19290462540783,
"currentReturnsPercentage": 9.618971219193973,
"remarks": "live data unavailable",
"xirr": 6.8782827063084975,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 28499.32,
"lastNavDate": "12-DEC-2022",
"bank": {
"accountNo": null,
"accountType": null,
"name": null,
"branch": null,
"city": null,
"pincode": null,
"micr": null,
"ifsc": null,
"neftifsc": null
},
"transactionFlags": {
"purAllow": null,
"redAllow": null,
"swtAllow": null,
"sipAllow": null,
"stpAllow": null,
"swpAllow": null
},
"lienUnitsFlag": null,
"availableUnits": null,
"lockInUnits": 0,
"availableAmount": null,
"mobile": null,
"taxStatus": null
}
],
"scheme": "Axis Bluechip Fund - Direct Growth",
"schemeCode": "EFDGG",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 575.2789664917238,
"nav": 49.54,
"currentValue": 28499.32,
"fundType": "NA",
"exitLoadRemarks": "NA",
"analytics": {
"investedValue": 25998.528979999995,
"averagePrice": 45.192907257759124,
"timestamp": "2023-02-02T12:24:56.413Z",
"realisedReturns": 0,
"currentReturns": 2500.791020000004,
"totalReturns": 2500.791020000004,
"currentReturnsPercentage": 9.618971219193973,
"sectorAllocationPercentage": [],
"remarks": "live data unavailable",
"schemeWeight": 37.620559762925765,
"xirr": 6.8782827063084975
}
}
],
"portfolio": {
"xirr": 9.774546962477466,
"schemeCount": 2,
"realisedReturns": 0,
"totalReturns": 9257.883992199997,
"currentReturns": 9257.883992199997,
"sectorAllocationPercentage": []
},
"exited": [
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"schemeCode": "D069G",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"assetType": "DEBT",
"brokerName": "INZ000208032",
"brokerCode": "INZ000208032",
"entryLoad": null,
"exitLoad": null,
"isDemat": "N",
"currentValue": 0,
"units": 0,
"nav": 44.5598,
"navUpdatedDate": "2022-11-09T18:30:00.000Z",
"avgBuyNav": null,
"returns": null,
"returnsPercent": null,
"xirr": null,
"sipFrequency": null,
"nextSipDate": null,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 0,
"lastNavDate": "12-DEC-2022"
}
]
},
"transactions": [
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-08-11T18:30:00.000Z",
"postedDate": "2020-08-11T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 29.743,
"purchasePrice": 33.62,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-13T18:30:00.000Z",
"postedDate": "2020-08-13T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE -",
"trxnAmount": 1499.93,
"trxnUnits": 9.663,
"purchasePrice": 155.2242,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-14T18:30:00.000Z",
"postedDate": "2020-08-14T18:30:00.000Z",
"trxnDescription": "Change / Regn of Nominee",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-16T18:30:00.000Z",
"postedDate": "2020-08-16T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-16T18:30:00.000Z",
"postedDate": "2020-08-16T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-30T18:30:00.000Z",
"postedDate": "2020-08-30T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-30T18:30:00.000Z",
"postedDate": "2020-08-30T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-09-14T18:30:00.000Z",
"postedDate": "2020-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 9.406,
"purchasePrice": 159.4651,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-10-04T18:30:00.000Z",
"postedDate": "2020-10-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 29.119,
"purchasePrice": 34.34,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-10-14T18:30:00.000Z",
"postedDate": "2020-10-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 9.73,
"purchasePrice": 154.1608,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-11-04T18:30:00.000Z",
"postedDate": "2020-11-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 27.418,
"purchasePrice": 36.47,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2020-11-16T18:30:00.000Z",
"postedDate": "2020-11-16T18:30:00.000Z",
"trxnDescription": "PURCHASE-BSE -",
"trxnAmount": 19999,
"trxnUnits": 486.054,
"purchasePrice": 41.1456,
"stampDuty": 1,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-11-16T18:30:00.000Z",
"postedDate": "2020-11-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 8.652,
"purchasePrice": 173.3543,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-12-06T18:30:00.000Z",
"postedDate": "2020-12-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 25.175,
"purchasePrice": 39.72,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-12-14T18:30:00.000Z",
"postedDate": "2020-12-14T18:30:00.000Z",
"trxnDescription": "Invalid Purchase15-DEC-2020_(Reversal - Code 1241)",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-12-15T18:30:00.000Z",
"postedDate": "2020-12-15T18:30:00.000Z",
"trxnDescription": "Purchase-BSE -",
"trxnAmount": 1499.93,
"trxnUnits": 8.023,
"purchasePrice": 186.9596,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "PURCHASE"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-01-04T18:30:00.000Z",
"postedDate": "2021-01-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.402,
"purchasePrice": 42.73,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-01-14T18:30:00.000Z",
"postedDate": "2021-01-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.832,
"purchasePrice": 191.5116,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-02-07T18:30:00.000Z",
"postedDate": "2021-02-07T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.705,
"purchasePrice": 44.04,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-02-14T18:30:00.000Z",
"postedDate": "2021-02-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.331,
"purchasePrice": 204.593,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2021-02-21T18:30:00.000Z",
"postedDate": "2021-02-21T18:30:00.000Z",
"trxnDescription": "Redemption(NEFT PUNJAB NATIONAL BANK)-BSE -",
"trxnAmount": -18152.82,
"trxnUnits": -447.17,
"purchasePrice": 40.5949,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2021-02-21T18:30:00.000Z",
"postedDate": "2021-02-21T18:30:00.000Z",
"trxnDescription": "Redemption(NEFT PUNJAB NATIONAL BANK)-BSE -",
"trxnAmount": -1602.53,
"trxnUnits": -38.884,
"purchasePrice": 41.2131,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-03-04T18:30:00.000Z",
"postedDate": "2021-03-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.94,
"purchasePrice": 43.59,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-03-14T18:30:00.000Z",
"postedDate": "2021-03-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.362,
"purchasePrice": 203.7451,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-04-04T18:30:00.000Z",
"postedDate": "2021-04-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.539,
"purchasePrice": 42.48,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-04-14T18:30:00.000Z",
"postedDate": "2021-04-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.422,
"purchasePrice": 202.0831,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-05-04T18:30:00.000Z",
"postedDate": "2021-05-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.363,
"purchasePrice": 42.8,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-05-16T18:30:00.000Z",
"postedDate": "2021-05-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.222,
"purchasePrice": 207.6756,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-06-06T18:30:00.000Z",
"postedDate": "2021-06-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.914,
"purchasePrice": 45.63,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-06-14T18:30:00.000Z",
"postedDate": "2021-06-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.59,
"purchasePrice": 227.5969,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-07-04T18:30:00.000Z",
"postedDate": "2021-07-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.421,
"purchasePrice": 46.68,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-07-14T18:30:00.000Z",
"postedDate": "2021-07-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.43,
"purchasePrice": 233.2793,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-08-04T18:30:00.000Z",
"postedDate": "2021-08-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.75,
"purchasePrice": 48.19,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-08-15T18:30:00.000Z",
"postedDate": "2021-08-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.268,
"purchasePrice": 239.2977,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-09-05T18:30:00.000Z",
"postedDate": "2021-09-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.3,
"purchasePrice": 51.81,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-09-14T18:30:00.000Z",
"postedDate": "2021-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.785,
"purchasePrice": 259.2935,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-10-04T18:30:00.000Z",
"postedDate": "2021-10-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.079,
"purchasePrice": 52.41,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-10-17T18:30:00.000Z",
"postedDate": "2021-10-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.535,
"purchasePrice": 271.0118,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-11-07T18:30:00.000Z",
"postedDate": "2021-11-07T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 18.701,
"purchasePrice": 53.47,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-11-14T18:30:00.000Z",
"postedDate": "2021-11-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.286,
"purchasePrice": 283.7797,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-12-05T18:30:00.000Z",
"postedDate": "2021-12-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.951,
"purchasePrice": 50.12,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-12-14T18:30:00.000Z",
"postedDate": "2021-12-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 17/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.527,
"purchasePrice": 271.3799,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-01-06T18:30:00.000Z",
"postedDate": "2022-01-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 18.888,
"purchasePrice": 52.94,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-01-16T18:30:00.000Z",
"postedDate": "2022-01-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 18/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.457,
"purchasePrice": 274.8835,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-02-06T18:30:00.000Z",
"postedDate": "2022-02-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.099,
"purchasePrice": 49.75,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-02-14T18:30:00.000Z",
"postedDate": "2022-02-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 19/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.861,
"purchasePrice": 255.9048,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-03-06T18:30:00.000Z",
"postedDate": "2022-03-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.838,
"purchasePrice": 45.79,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-03-14T18:30:00.000Z",
"postedDate": "2022-03-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 20/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.091,
"purchasePrice": 246.2527,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-04-04T18:30:00.000Z",
"postedDate": "2022-04-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.622,
"purchasePrice": 50.96,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-04-17T18:30:00.000Z",
"postedDate": "2022-04-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 21/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.893,
"purchasePrice": 254.5333,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-05-04T18:30:00.000Z",
"postedDate": "2022-05-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.353,
"purchasePrice": 46.83,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-05-15T18:30:00.000Z",
"postedDate": "2022-05-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 22/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.473,
"purchasePrice": 231.7365,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-06-05T18:30:00.000Z",
"postedDate": "2022-06-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.886,
"purchasePrice": 45.69,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-06-14T18:30:00.000Z",
"postedDate": "2022-06-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 23/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.68,
"purchasePrice": 224.5359,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-07-05T18:30:00.000Z",
"postedDate": "2022-07-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.325,
"purchasePrice": 44.79,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-07-17T18:30:00.000Z",
"postedDate": "2022-07-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 24/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.338,
"purchasePrice": 236.6454,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-08-04T18:30:00.000Z",
"postedDate": "2022-08-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.378,
"purchasePrice": 49.07,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-08-15T18:30:00.000Z",
"postedDate": "2022-08-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 25/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.894,
"purchasePrice": 254.4763,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-09-14T18:30:00.000Z",
"postedDate": "2022-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 26/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.777,
"purchasePrice": 259.6198,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-10-05T18:30:00.000Z",
"postedDate": "2022-10-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.411,
"purchasePrice": 48.99,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-10-16T18:30:00.000Z",
"postedDate": "2022-10-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 27/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.946,
"purchasePrice": 252.2704,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-11-06T18:30:00.000Z",
"postedDate": "2022-11-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.959,
"purchasePrice": 50.1,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
}
],
"userInfo": {
"pan": "XXXXX",
"name": "MR.XXXX XXX",
"phone": "9008633421",
"email": "[email protected]",
"address": {
"address1": "XXX XX XX",
"address2": "",
"address3": "",
"city": "XX",
"district": "",
"state": "XX",
"pincode": "XXXX",
"country": "India"
},
"dpIds": null,
"bankAccounts": null,
"kycStatus": "04",
"taxStatus": null,
"emails": [
"[email protected]"
]
}
}
{
"fromDate": "1-Jan-1986",
"toDate": "7-Dec-2022 ",
"snapshotDate": "2022-12-07T12:21:04.934Z",
"transactionId": "TRX_cd0ca32353b14f6b92bcd0dfb0b05c32",
"gateway": "gatewaydemo",
"timestamp": "2025-01-01T12:00:00.000Z",
"checksum": "a604cc4e0a4b99a027cd1782c2551e33aab3fbc6a712797c29b8c4b555f9e529",
"summary": {
"schemeDetails": [
{
"isin": "INF200K01RJ1",
"folios": [
{
"folioNumber": "xxxxxxxx",
"brokerName": "INZ000208032",
"brokerCode": "INZ000208032",
"isDemat": "N",
"units": 184.47399999999996,
"investedValue": 40498.228052800005,
"currentValue": 47255.321025,
"currentReturns": 6757.092972199993,
"totalReturns": 6757.092972199993,
"realisedReturns": 0,
"averagePrice": 219.53352804622875,
"currentReturnsPercentage": 16.684910172836105,
"remarks": "live data unavailable",
"xirr": 11.577337973980805,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 47255.321025,
"lastNavDate": "12-DEC-2022",
"bank": {
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
},
"transactionFlags": {
"purAllow": "Y",
"swtAllow": "Y",
"redAllow": "Y",
"sipAllow": "Y",
"stpAllow": "Y",
"swpAllow": "Y"
},
"lienUnitsFlag": "N",
"lockInUnits": 0,
"availableUnits": 123,
"availableAmount": 12344,
"mobile": "9XXXXXXXXX",
"taxStatus": "01",
"dpId": "XXXXX"
}
],
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"schemeCode": "D81G",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 184.47399999999996,
"nav": 256.1625,
"currentValue": 47255.321025,
"fundType": "NA",
"exitLoadRemarks": "NA",
"analytics": {
"investedValue": 40498.228052800005,
"averagePrice": 219.5335280462288,
"timestamp": "2023-02-02T12:24:56.413Z",
"realisedReturns": 0,
"currentReturns": 6757.092972199993,
"totalReturns": 6757.092972199993,
"currentReturnsPercentage": 16.684910172836105,
"sectorAllocationPercentage": [],
"remarks": "live data unavailable",
"schemeWeight": 62.37944023707425,
"xirr": 11.577337973980805
}
},
{
"isin": "INF846K01DP8",
"folios": [
{
"folioNumber": "abc12345",
"brokerName": "DIRECT",
"brokerCode": "INZ000208032",
"isDemat": "N",
"units": 575.2789664917238,
"investedValue": 25998.528979999995,
"currentValue": 28499.32,
"currentReturns": 2500.791020000004,
"totalReturns": 2500.791020000004,
"realisedReturns": 0,
"averagePrice": 45.19290462540783,
"currentReturnsPercentage": 9.618971219193973,
"remarks": "live data unavailable",
"xirr": 6.8782827063084975,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 28499.32,
"lastNavDate": "12-DEC-2022",
"bank": {
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
},
"transactionFlags": {
"purAllow": "Y",
"swtAllow": "Y",
"redAllow": "Y",
"sipAllow": "Y",
"stpAllow": "Y",
"swpAllow": "Y"
},
"lienUnitsFlag": "N",
"lockInUnits": 0,
"availableUnits": 123,
"availableAmount": 12344,
"mobile": "9XXXXXXXXX",
"taxStatus": "01",
"dpId": "XXXXX"
}
],
"scheme": "Axis Bluechip Fund - Direct Growth",
"schemeCode": "EFDGG",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 575.2789664917238,
"nav": 49.54,
"currentValue": 28499.32,
"fundType": "NA",
"exitLoadRemarks": "NA",
"analytics": {
"investedValue": 25998.528979999995,
"averagePrice": 45.192907257759124,
"timestamp": "2023-02-02T12:24:56.413Z",
"realisedReturns": 0,
"currentReturns": 2500.791020000004,
"totalReturns": 2500.791020000004,
"currentReturnsPercentage": 9.618971219193973,
"sectorAllocationPercentage": [],
"remarks": "live data unavailable",
"schemeWeight": 37.620559762925765,
"xirr": 6.8782827063084975
}
}
],
"portfolio": {
"xirr": 9.774546962477466,
"schemeCount": 2,
"realisedReturns": 0,
"totalReturns": 9257.883992199997,
"currentReturns": 9257.883992199997,
"sectorAllocationPercentage": []
},
"exited": [
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"schemeCode": "D069G",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"assetType": "DEBT",
"brokerName": "INZ000208032",
"brokerCode": "INZ000208032",
"entryLoad": null,
"exitLoad": null,
"isDemat": "N",
"currentValue": 0,
"units": 0,
"nav": 44.5598,
"navUpdatedDate": "2022-11-09T18:30:00.000Z",
"avgBuyNav": null,
"returns": null,
"returnsPercent": null,
"xirr": null,
"sipFrequency": null,
"nextSipDate": null,
"rtaCode": "KFINTECH",
"kycStatus": "3",
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 3,
"lastTrxnDate": "12-DEC-2022",
"openingBal": 0,
"closingBal": 0,
"lastNavDate": "12-DEC-2022"
}
]
},
"transactions": [
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-08-11T18:30:00.000Z",
"postedDate": "2020-08-11T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 29.743,
"purchasePrice": 33.62,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-13T18:30:00.000Z",
"postedDate": "2020-08-13T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE -",
"trxnAmount": 1499.93,
"trxnUnits": 9.663,
"purchasePrice": 155.2242,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-14T18:30:00.000Z",
"postedDate": "2020-08-14T18:30:00.000Z",
"trxnDescription": "Change / Regn of Nominee",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-16T18:30:00.000Z",
"postedDate": "2020-08-16T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-16T18:30:00.000Z",
"postedDate": "2020-08-16T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-30T18:30:00.000Z",
"postedDate": "2020-08-30T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-08-30T18:30:00.000Z",
"postedDate": "2020-08-30T18:30:00.000Z",
"trxnDescription": "Address Updated from KRA Data",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-09-14T18:30:00.000Z",
"postedDate": "2020-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 9.406,
"purchasePrice": 159.4651,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-10-04T18:30:00.000Z",
"postedDate": "2020-10-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 29.119,
"purchasePrice": 34.34,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-10-14T18:30:00.000Z",
"postedDate": "2020-10-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 9.73,
"purchasePrice": 154.1608,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-11-04T18:30:00.000Z",
"postedDate": "2020-11-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 27.418,
"purchasePrice": 36.47,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2020-11-16T18:30:00.000Z",
"postedDate": "2020-11-16T18:30:00.000Z",
"trxnDescription": "PURCHASE-BSE -",
"trxnAmount": 19999,
"trxnUnits": 486.054,
"purchasePrice": 41.1456,
"stampDuty": 1,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-11-16T18:30:00.000Z",
"postedDate": "2020-11-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 8.652,
"purchasePrice": 173.3543,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2020-12-06T18:30:00.000Z",
"postedDate": "2020-12-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 25.175,
"purchasePrice": 39.72,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-12-14T18:30:00.000Z",
"postedDate": "2020-12-14T18:30:00.000Z",
"trxnDescription": "Invalid Purchase15-DEC-2020_(Reversal - Code 1241)",
"trxnAmount": 0,
"trxnUnits": 0,
"purchasePrice": 0,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2020-12-15T18:30:00.000Z",
"postedDate": "2020-12-15T18:30:00.000Z",
"trxnDescription": "Purchase-BSE -",
"trxnAmount": 1499.93,
"trxnUnits": 8.023,
"purchasePrice": 186.9596,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "PURCHASE"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-01-04T18:30:00.000Z",
"postedDate": "2021-01-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.402,
"purchasePrice": 42.73,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-01-14T18:30:00.000Z",
"postedDate": "2021-01-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.832,
"purchasePrice": 191.5116,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-02-07T18:30:00.000Z",
"postedDate": "2021-02-07T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.705,
"purchasePrice": 44.04,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-02-14T18:30:00.000Z",
"postedDate": "2021-02-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.331,
"purchasePrice": 204.593,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2021-02-21T18:30:00.000Z",
"postedDate": "2021-02-21T18:30:00.000Z",
"trxnDescription": "Redemption(NEFT PUNJAB NATIONAL BANK)-BSE -",
"trxnAmount": -18152.82,
"trxnUnits": -447.17,
"purchasePrice": 40.5949,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01VB0",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D069G",
"scheme": "SBI Magnum Medium Duration Fund Direct Growth (formerly SBI Regular Savings Fund)",
"transactionDate": "2021-02-21T18:30:00.000Z",
"postedDate": "2021-02-21T18:30:00.000Z",
"trxnDescription": "Redemption(NEFT PUNJAB NATIONAL BANK)-BSE -",
"trxnAmount": -1602.53,
"trxnUnits": -38.884,
"purchasePrice": 41.2131,
"stampDuty": 0,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": true,
"transactionType": "NA"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-03-04T18:30:00.000Z",
"postedDate": "2021-03-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.94,
"purchasePrice": 43.59,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-03-14T18:30:00.000Z",
"postedDate": "2021-03-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.362,
"purchasePrice": 203.7451,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-04-04T18:30:00.000Z",
"postedDate": "2021-04-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.539,
"purchasePrice": 42.48,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-04-14T18:30:00.000Z",
"postedDate": "2021-04-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.422,
"purchasePrice": 202.0831,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-05-04T18:30:00.000Z",
"postedDate": "2021-05-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 23.363,
"purchasePrice": 42.8,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-05-16T18:30:00.000Z",
"postedDate": "2021-05-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 7.222,
"purchasePrice": 207.6756,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-06-06T18:30:00.000Z",
"postedDate": "2021-06-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.914,
"purchasePrice": 45.63,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-06-14T18:30:00.000Z",
"postedDate": "2021-06-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.59,
"purchasePrice": 227.5969,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-07-04T18:30:00.000Z",
"postedDate": "2021-07-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.421,
"purchasePrice": 46.68,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-07-14T18:30:00.000Z",
"postedDate": "2021-07-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.43,
"purchasePrice": 233.2793,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-08-04T18:30:00.000Z",
"postedDate": "2021-08-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.75,
"purchasePrice": 48.19,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-08-15T18:30:00.000Z",
"postedDate": "2021-08-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.268,
"purchasePrice": 239.2977,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-09-05T18:30:00.000Z",
"postedDate": "2021-09-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.3,
"purchasePrice": 51.81,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-09-14T18:30:00.000Z",
"postedDate": "2021-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.785,
"purchasePrice": 259.2935,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-10-04T18:30:00.000Z",
"postedDate": "2021-10-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.079,
"purchasePrice": 52.41,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-10-17T18:30:00.000Z",
"postedDate": "2021-10-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.535,
"purchasePrice": 271.0118,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-11-07T18:30:00.000Z",
"postedDate": "2021-11-07T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 18.701,
"purchasePrice": 53.47,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-11-14T18:30:00.000Z",
"postedDate": "2021-11-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE --Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.286,
"purchasePrice": 283.7797,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2021-12-05T18:30:00.000Z",
"postedDate": "2021-12-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.951,
"purchasePrice": 50.12,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2021-12-14T18:30:00.000Z",
"postedDate": "2021-12-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 17/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.527,
"purchasePrice": 271.3799,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-01-06T18:30:00.000Z",
"postedDate": "2022-01-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 18.888,
"purchasePrice": 52.94,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-01-16T18:30:00.000Z",
"postedDate": "2022-01-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 18/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.457,
"purchasePrice": 274.8835,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-02-06T18:30:00.000Z",
"postedDate": "2022-02-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.099,
"purchasePrice": 49.75,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-02-14T18:30:00.000Z",
"postedDate": "2022-02-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 19/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.861,
"purchasePrice": 255.9048,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-03-06T18:30:00.000Z",
"postedDate": "2022-03-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.838,
"purchasePrice": 45.79,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-03-14T18:30:00.000Z",
"postedDate": "2022-03-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 20/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.091,
"purchasePrice": 246.2527,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-04-04T18:30:00.000Z",
"postedDate": "2022-04-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.622,
"purchasePrice": 50.96,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-04-17T18:30:00.000Z",
"postedDate": "2022-04-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 21/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.893,
"purchasePrice": 254.5333,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-05-04T18:30:00.000Z",
"postedDate": "2022-05-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.353,
"purchasePrice": 46.83,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-05-15T18:30:00.000Z",
"postedDate": "2022-05-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 22/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.473,
"purchasePrice": 231.7365,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-06-05T18:30:00.000Z",
"postedDate": "2022-06-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 21.886,
"purchasePrice": 45.69,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-06-14T18:30:00.000Z",
"postedDate": "2022-06-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 23/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.68,
"purchasePrice": 224.5359,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-07-05T18:30:00.000Z",
"postedDate": "2022-07-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 22.325,
"purchasePrice": 44.79,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-07-17T18:30:00.000Z",
"postedDate": "2022-07-17T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 24/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 6.338,
"purchasePrice": 236.6454,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-08-04T18:30:00.000Z",
"postedDate": "2022-08-04T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.378,
"purchasePrice": 49.07,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-08-15T18:30:00.000Z",
"postedDate": "2022-08-15T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 25/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.894,
"purchasePrice": 254.4763,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-09-14T18:30:00.000Z",
"postedDate": "2022-09-14T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 26/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.777,
"purchasePrice": 259.6198,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-10-05T18:30:00.000Z",
"postedDate": "2022-10-05T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 20.411,
"purchasePrice": 48.99,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF200K01RJ1",
"folioNumber": "xxxxxxxx",
"amc": "SBI Mutual Fund",
"amcCode": "L",
"schemeCode": "D81G",
"scheme": "SBI Focused Equity Fund Direct Growth (formerly SBI Emerging Businesses Fund)",
"transactionDate": "2022-10-16T18:30:00.000Z",
"postedDate": "2022-10-16T18:30:00.000Z",
"trxnDescription": "Purchase - Systematic-BSE - Instalment No - 27/1000-Exchange",
"trxnAmount": 1499.93,
"trxnUnits": 5.946,
"purchasePrice": 252.2704,
"stampDuty": 0.07,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
},
{
"isin": "INF846K01DP8",
"folioNumber": "abc12345",
"amc": "AXIS Mutual Fund",
"amcCode": "128",
"schemeCode": "EFDGG",
"scheme": "Axis Bluechip Fund - Direct Growth",
"transactionDate": "2022-11-06T18:30:00.000Z",
"postedDate": "2022-11-06T18:30:00.000Z",
"trxnDescription": "Systematic Investment (1)",
"trxnAmount": 999.95,
"trxnUnits": 19.959,
"purchasePrice": 50.1,
"stampDuty": 0.05,
"totalTax": 0,
"transactionMode": "N",
"cashFlow": null,
"analytics": {
"exited": false,
"transactionType": "DEPOSIT",
"transactionStatus": "SUCCESS",
"transactionDescription": "SIP"
},
"checkDigit": 0,
"email": "[email protected]",
"trxnCharge": 0,
"sttTax": 0,
"tax": 0
}
],
"userInfo": {
"pan": "XXXXX",
"name": "MR.XXXX XXX",
"phone": "9008633421",
"email": "[email protected]",
"address": {
"address1": "XXX XX XX",
"address2": "",
"address3": "",
"city": "XX",
"district": "",
"state": "XX",
"pincode": "XXXX",
"country": "India"
},
"dpIds": [
"120816XXXX31086"
],
"bankAccounts": [
{
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
}
],
"kycStatus": "04",
"taxStatus": "01",
"emails": [
"[email protected]"
]
}
}
{
"summary": {
"folioCount": 3,
"exited": [],
"schemeDetails": [
{
"isin": "INF109K012K1",
"folios": [
{
"folioNumber": "xxxxxxxx",
"brokerName": "INZ000208032",
"brokerCode": "INZ000208032",
"isDemat": "N",
"units": 3.89,
"rtaCode": "CAMS",
"kycStatus": null,
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 2,
"lastTrxnDate": null,
"openingBal": null,
"closingBalance": 3.89,
"lastNavDate": "08-Nov-2023",
"bank": {
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
},
"transactionFlags": {
"purAllow": "Y",
"swtAllow": "Y",
"redAllow": "Y",
"sipAllow": "Y",
"stpAllow": "Y",
"swpAllow": "Y"
},
"lienUnitsFlag": "N",
"availableUnits": "3.889",
"availableAmount": "1390.98",
"taxStatus": "01",
"investedValue": 1000,
"currentReturns": 390.98,
"currentReturnsPercentage": 39.1,
"totalReturns": null,
"currentValue": 1390.98,
"realisedReturns": null,
"averagePrice": null,
"xirr": null,
"mobile": "+9194XXXXXXXX",
"dpId": "XXXX"
}
],
"scheme": "ICICI Prudential Value Discovery Fund - Direct Plan - Growth",
"schemeCode": "8176",
"amc": "ICICI Prudential Mutual Fund",
"amcCode": "P",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 3.89,
"nav": 357.67,
"fundType": null,
"exitLoadRemarks": null,
"analytics": null,
"currentValue": 1390.98
},
{
"isin": "INF879O01027",
"folios": [
{
"folioNumber": "abc12345",
"brokerName": "ARN-DIRECT",
"brokerCode": "DIRECT",
"isDemat": "N",
"units": 81.76,
"rtaCode": "CAMS",
"kycStatus": null,
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 4,
"lastTrxnDate": null,
"openingBal": null,
"closingBalance": 81.76,
"lastNavDate": "08-Nov-2023",
"bank": {
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
},
"transactionFlags": {
"purAllow": "Y",
"swtAllow": "Y",
"redAllow": "Y",
"sipAllow": "Y",
"stpAllow": "Y",
"swpAllow": "Y"
},
"lienUnitsFlag": "N",
"availableUnits": "81.760",
"availableAmount": "5272.42",
"taxStatus": "01",
"investedValue": 5000,
"currentReturns": 272.42,
"currentReturnsPercentage": 5.45,
"totalReturns": null,
"currentValue": 5272.42,
"realisedReturns": null,
"averagePrice": null,
"xirr": null,
"mobile": "+919XXXXXXXXX",
"dpId": ""
},
{
"folioNumber": "13664121",
"brokerName": "Arevuk Advisory Services Private Ltd",
"brokerCode": "INA200005166",
"isDemat": "N",
"units": 413.18,
"rtaCode": "CAMS",
"kycStatus": null,
"email": "[email protected]",
"decimalUnits": 3,
"decimalAmount": 2,
"decimalNav": 4,
"lastTrxnDate": null,
"openingBal": null,
"closingBalance": 413.18,
"lastNavDate": "08-Nov-2023",
"bank": {
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
},
"transactionFlags": {
"purAllow": "Y",
"swtAllow": "Y",
"redAllow": "Y",
"sipAllow": "Y",
"stpAllow": "Y",
"swpAllow": "Y"
},
"lienUnitsFlag": "N",
"availableUnits": "413.183",
"availableAmount": "26644.77",
"taxStatus": "01",
"investedValue": 25000,
"currentReturns": 1644.77,
"currentReturnsPercentage": 6.58,
"totalReturns": null,
"currentValue": 26644.77,
"realisedReturns": null,
"averagePrice": null,
"xirr": null,
"mobile": "+919XXXXXXXXX",
"dpId": ""
}
],
"scheme": "Parag Parikh Flexi Cap Fund - Direct Plan",
"schemeCode": "001ZG",
"amc": "PPFAS Mutual Fund",
"amcCode": "PP",
"assetType": "EQUITY",
"entryLoad": null,
"exitLoad": null,
"units": 494.94,
"nav": 64.4866,
"fundType": null,
"exitLoadRemarks": null,
"analytics": null,
"currentValue": 31917.190000000002
}
],
"portfolio": {}
},
"transactions": null,
"userInfo": {
"pan": "XXXXX",
"name": "MR.XXXX XXX",
"phone": "9008633421",
"email": "[email protected]",
"address": {
"address1": "XXX XX XX",
"address2": "",
"address3": "",
"city": "XX",
"district": "",
"state": "XX",
"pincode": "XXXX",
"country": "India"
},
"dpIds": [
"12081600XXXXX"
],
"bankAccounts": [
{
"accountNo": "XXXX",
"accountType": "XX",
"name": "XX XX OF INDIA",
"branch": "XX",
"city": "XX",
"pincode": "",
"micr": "",
"ifsc": "XX",
"neftifsc": "XX"
}
],
"kycStatus": null,
"taxStatus": "01",
"emails": null
},
"snapshotDate": "2023-11-09T12:41:29.200Z",
"fromDate": null,
"toDate": null,
"transactionId": "TRX_787eff1d62504fd28be47a7e839be8f4z16",
"gateway": "gatewaydemo-stag",
"timestamp": "2023-11-09T12:41:29.227Z",
"checksum": "44b2eb4a8f6160d98fbfe8ade9df238aeaf2138fcad09281afb972d83358bee9"
}
/* When MFC could not share holdings in certain duration */
{
"transactionId" : "TRX_c21de8230c484a85b14084c5a4e23b17",
"error" : {
"value" : true,
"message" : "mfc_timeout",
"code" : 2003
},
"timestamp" : "2025-01-01T12:00:00.000Z",
"checksum" : "e6d262105b897f0560b4d00907013755fc5360fad54e9e90a43453803af4ef47"
}
/* When MFC API shares unexpected response when smallcase requests for holdings */
{
"transactionId" : "TRX_2a7de8230c484a8eb4084c5a4e401s21",
"error" : {
"value" : true,
"message" : "mfc_bad_response",
"code" : 2004
},
"timestamp" : "2025-01-01T12:00:00.000Z",
"checksum" : "e6d262105b897f0560b4d00907013755fc5360fad54e9e90a43453803af4ef47"
}
/* When unexpected condition is encountered */
{
"transactionId" : "TRX_c34de8230c484a8s24084c5a4e493a22",
"error" : {
"value" : true,
"message" : "internal_error",
"code" : 2001
},
"timestamp" : "2025-01-01T12:00:00.000Z",
"checksum" : "e6d262105b897f0560b4d00907013755fc5360fad54e9e90a43453803af4ef47"
}
/* When MFC could not share holdings in certain duration */
{
"transactionId" : "TRX_c21de8230c484a85b14084c5a4e23b17",
"error" : {
"value" : true,
"message" : "mfc_timeout",
"code" : 2003
},
"timestamp" : "2025-01-01T12:00:00.000Z",
"checksum" : "e6d262105b897f0560b4d00907013755fc5360fad54e9e90a43453803af4ef47"
}
Implementation steps
Step 1 - Install SDK
To load/install the SDK, checkout the installation step on the respective SDK integration page:
-
JavaScript SDK
-
Android SDK
-
iOS SDK
-
Flutter SDK
-
React Native SDK
-
Cordova SDK
Step 2 - Initialise gateway session
Next up, you need to initialise the gateway session on SDK (using guest user auth token). Check:
- Init on JavaScript SDK
- Init on Android SDK
- Init on iOS SDK
- Init on Flutter SDK
- Init on React Native SDK
- Init on Cordova SDK
Step 3 - Create transaction on your server
Create a transaction ID by calling gateway API from your server.
Reference: Mutual Fund Holdings Import - Create TransactionId
Step 4 - Trigger MF transaction on SDK
gatewayInstance.triggerMfTransaction({
// ! Use valid transactionId with intent MF_HOLDINGS_IMPORT
transactionId: "<transactionId>"
})
.then(txnResponse => {
/*
* Holdings import complete. Holdings data should be hit to registered webhook
*/
console.log('received response:', txnResponse);
})
.catch(err => {
/*
* User dropped before confirming holdings fetch
* Possible errors - https://developers.gateway.smallcase.com/docs/transaction-errors
*/
console.log('received error:', err.message);
});
SmallcaseGatewaySdk.triggerMfTransaction(
activity = $currentActivity,
transactionId = $transactionId, // ! Use valid transactionId with intent MF_HOLDINGS_IMPORT
object : MFHoldingsResponseListener {
override fun onSuccess(transactionResult: TransactionResult) {
/**
* Holdings import complete. Holdings data should be hit to registered webhook
*/
}
override fun onError(errorCode: Int, errorMessage: String, data: String?) {
/**
* User dropped before confirming holdings fetch
* Possible errors - https://developers.gateway.smallcase.com/docs/transaction-errors
*/
}
})
do {
try SCGateway.shared.triggerMfTransaction(
presentingController: $currentViewController,
transactionId: $transactionId, // ! Use valid transactionId with intent MF_HOLDINGS_IMPORT
completion: { (result) in
switch result {
case .success(let response):
/**
* Holdings import complete. Holdings data should be hit to registered webhook
*/
case .failure(let error):
/**
* User dropped before confirming holdings fetch
* Possible errors - https://developers.gateway.smallcase.com/docs/transaction-errors
*/
}
})
}
catch let err {
/**
* Gateway SDK is not initialized
*/
}
try {
// ! Use valid transactionId with intent MF_HOLDINGS_IMPORT
final String? response = await ScgatewayFlutterPlugin.triggerMfGatewayTransaction($transactionId);
/**
* Holdings import complete. Holdings data should be hit to registered webhook
*/
} on Exception catch(e) {
/**
* User dropped before confirming holdings fetch
* Possible errors - https://developers.gateway.smallcase.com/docs/transaction-errors
*/
}
Analytics
smallcase Gateway also provides analytics in the webhook response as described in the above response structure for each and every import done by the user
One can also get updated analytics periodically by using the same holdings import snapshot using the Analytics API.
Updated 6 days ago