smallcase Module Integration
A plug and play solution for smallcase transactions enabling distributors (& creators) to offer smallcases to their captive audience.
Prerequisites reads
Implementation steps
Step 1 - Install SDK
To load JS SDK, or install and configure native SDK, checkout the installation step on the respective SDK integration page:
-
JavaScript SDK
-
Android SDK
-
iOS SDK
-
Flutter SDK
-
React Native SDK
-
Cordova SDK
Step 2 - Initialise gateway session
Next up, you need to initialise the gateway session on SDK. Check:
- Init on JavaScript SDK
- Init on Android SDK
- Init on iOS SDK
- Init on Flutter SDK
- Init on React Native SDK
- Init on Cordova SDK
Step 3 - Launch smallcase module (aka smallplug)
gatewayInstance
.openSmallplug()
.then(res => console.log('smallcase module closed with following response:', res);
SmallcaseGatewaySdk.launchSmallPlug(
activity: Activity, //example: requireActivity()
smallplugData: SmallplugData?,
smallPlugListener = object : SmallPlugResponseListener {
override fun onSuccess(smallPlugResult: SmallPlugResult) {
//success callback
}
override fun onFailure(errorCode: Int, errorMessage: String) {
//error callback
}
},
smallplugPartnerProps: SmallplugPartnerProps?
class SmallplugData(
val targetEndpoint: String?, //example: "discovery/all"
val params: String? //example: "count=11&minInvest=0-5000"
)
class SmallplugPartnerProps(
headerColor: String?, //example: "2C3639"
headerOpacity: Double, //example: 1.0
backIconColor: String?, //example: "2C3639"
backIconOpacity: Double? //example: 1.0
)
SCGateway.shared.launchSmallPlug(
presentingController: UIViewController, //example: self
smallplugData: SmallplugData?,
smallplugUiConfig: SmallplugUiConfig? { (response, error) in
if(response != nil) {
//success callback
} else {
//error callback
}
}
class SmallplugData: NSObject {
targetEndpoint: String? //example: "discovery/all"
params: String? //example: "count=11&minInvest=0-5000"
}
class SmallplugUiConfig: NSObject {
headerColor: String? //example: "2C3639"
backIconColor: String? //example: "2C3639"
opacity: CGFloat? //example: 1.0
backIconColorOpacity: CGFloat? //example: 1.0
}
Future<String?> ScgatewayFlutterPlugin.launchSmallplugWithBranding(
SmallplugData smallplugData,
SmallplugUiConfig smallplugUiConfig
);
class SmallplugData {
String? targetEndpoint; //example: "discovery/all"
String? params; //example: "count=11&minInvest=0-5000"
}
class SmallplugUiConfig {
Color? headerColor; //example: Colors.black
double headerOpacity; //example: 1.0
Color? backIconColor; //example: Colors.white
double backIconOpacity; //example: 1.0
}
try {
const res = await SmallcaseGateway.launchSmallplugWithBranding(
targetEndpoint, //example: "discovery/all"
params, //example: "count=11&minInvest=0-5000"
headerColorHex, //example: "#2F363F"
headerOpacity, //example: 1.0
backIconColorHex, //example: "#2F363F"
backIconOpacity //example: 1.0
);
} catch(err) {
//error callback
}
scgateway.launchSmallplug(function(data) {
// success callback
}, function(error) {
// error callback
}, [
targetEndpoint, //example: "discovery/all"
params, //example: "count=11&minInvest=0-5000"
headerColorHex, //example: "#2F363F"
headerOpacity, //example: 1.0
backIconColorHex, //example: "#2F363F"
backIconOpacity //example: 1.0
]);
This will launch the smallcase distribution user-interface where a user would be able to place a smallcase order.
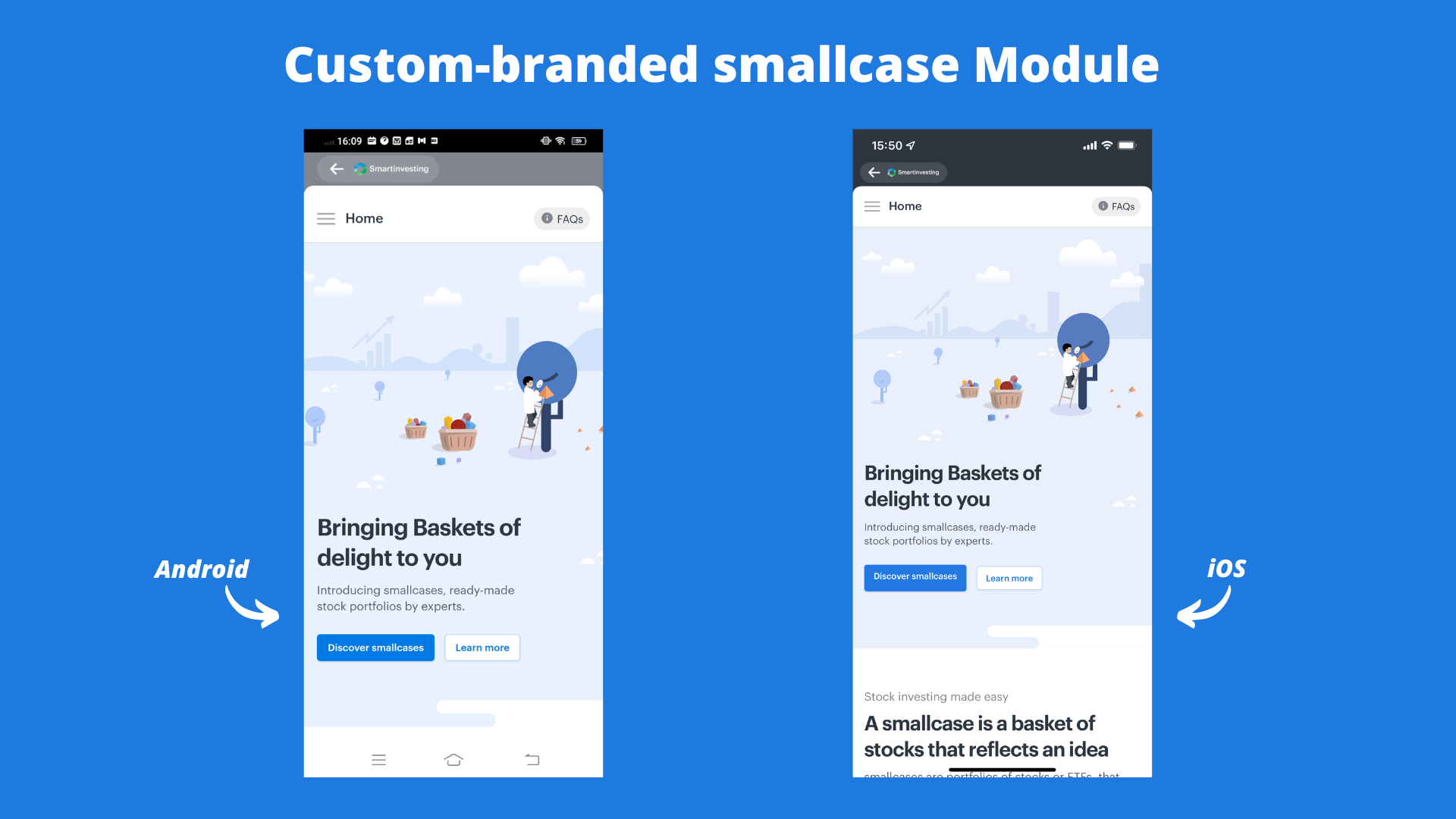
Your brand logo is required for custom branding
Share the PNG logo of your brand with us, if not already shared by your team:
SDK Height Width Remark Web 40px 98 to 240px monochrome color preferred (to be used over white bg) Mobile 16px 32 to 96px as per your brand color
Step 4 - Handle SDK response (on close of smallcase module)
When the user closes the smallcase module, the gateway SDK returns the following response -
/*
* if user logged in with broker before closing the smallcase module,
* connected user auth token is shared as `smallcaseAuthToken`.
*
* more about auth tokens: https://developers.gateway.smallcase.com/docs/getting-started#broker-identification
*/
{
"success": true,
"smallcaseAuthToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzbWFsbGNhc2VBdXRoSWQiOiI2MjUxMjY4ZDBlZjczZjJlM2I1M2UyZmEiLCJpYXQiOjE2NDk0ODU0NjksImV4cCI6MTY0OTQ4OTA2OX0.mHbSc7XFX0E-5vPx5l4BMPJC9Zv0b7wcjJmbMKmiwMQ"
}
/*
* if user closed the flow before broker login,
* guest auth token is shared as `smallcaseAuthToken`.
*
* more about auth tokens: https://developers.gateway.smallcase.com/docs/getting-started#broker-identification
*/
{
"success": true,
"smallcaseAuthToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJndWVzdCI6dHJ1ZX0.CqynDZ2nS3aTAF9Ohdj6TZ7PZbKiYhPw1WzGM6fgwFs"
}
/*
* if user logged in with broker before closing the smallcase module,
* connected user auth token is shared as `smallcaseAuthToken`.
*
* more about auth tokens: https://developers.gateway.smallcase.com/docs/getting-started#broker-identification
*/
{
"success": true,
"smallcaseAuthToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzbWFsbGNhc2VBdXRoSWQiOiI2MjUxMjY4ZDBlZjczZjJlM2I1M2UyZmEiLCJpYXQiOjE2NDk0ODU0NjksImV4cCI6MTY0OTQ4OTA2OX0.mHbSc7XFX0E-5vPx5l4BMPJC9Zv0b7wcjJmbMKmiwMQ"
}
/*
* if user closed the flow before broker login, this is the response -
*/
{
"success": true,
"smallcaseAuthToken": undefined
}
smallcaseAuthToken
is a (connected user auth token) used for user identification and returning user journeys (learn more).
FAQs
How can we display investment details in our UI before opening smallcase module?
While DM provides a ready-made UI which takes all possible user journeys, it is also possible to display some parts of the UI (list of smallcases, investment overview, etc) outside of the DM flow and directly in your app / website. You can use server-side data APIs to consume investment-related data and display it in your UI (outside of the smallcase Module).
How can we directly launch smallcase module on a page other than homepage?
Based on the user journey in your product, you might want to open smallcase module on some specific page. Say, users should view the discover page by default where all smallcase are listed. The same is possible by passing the URL endpoint & additional parameters (called URL params).
Pass relevant parameters in the function call to relevant smallplug method -
class SmallplugData {
String? targetEndpoint;
String? params;
}
SmallplugData smallplugData = new SmallplugData();
smallplugData.targetEndpoint = "discover/all";
smallplugData.params = "count=11&minInvest=0-5000";
ScgatewayFlutterPlugin.launchSmallplug(smallplugData)
class SmallplugData(
val targetEndpoint: String?,
val params: String?
)
SmallcaseGatewaySdk.launchSmallPlug(
requireActivity(),
SmallplugData(targetEndpoint = "discover/all", params = "count=11&minInvest=0-5000") ,
object : SmallPlugResponseListener {
override fun onSuccess(smallPlugResult: SmallPlugResult) {
//success callback
}
override fun onFailure(errorCode: Int, errorMessage: String) {
//error callback
}
}
)
@objcMembers public class SmallplugData: NSObject {
public var targetEndpoint: String?
public var params: String?
}
SCGateway.shared.launchSmallPlug(
presentingController: self,
smallplugData: SmallplugData(targetEndpoint: "discover/all", params: "count=11&minInvest=0-5000")
) { (response, error) in
if(response != nil) {
//success callback
} else {
//error callback
}
}
gatewayInsance.openSmallplug({
path: "discover/all",
params: null
});
This will launch the smallcase distribution user interface at the specified route -
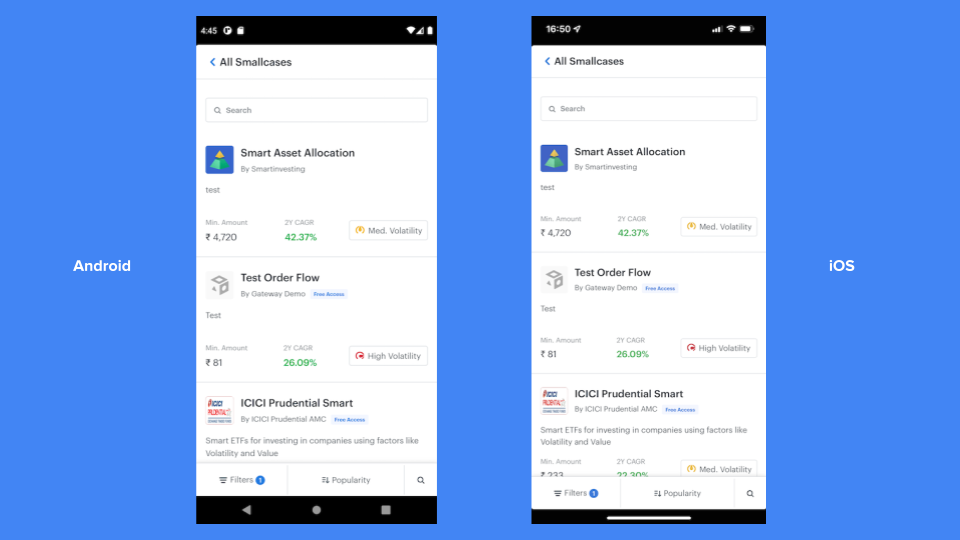
launching smallcase module with discover route as target endpoint
Supported endpoints
Endpoints | description |
---|---|
blank (default) | homepage |
discover/all | smallcase discovery page |
smallcase/<scid> | smallcase profile page displaying details about particular smallcase |
smallcase/<scid>/stocks | same as smallcase profile, but with "stocks & weights" tab selected by default |
details/<iscid> | investment details for an invested smallcase |
orders/<iscid> | orders related to an invested smallcase |
notifications | notifications page |
fees | fees page displaying fees deducted for each of their investment |
Note: some endpoints requires scid / iscid
Discover page: customize default smallcase filtering and sorting
discover
endpoint support multiple parameter to filter & sort smallcases by default.
additional parameters to filter & sort smallcases
Param for filtering | description | example |
---|---|---|
count | number of smallcase to display in first load | count=10& |
minInvest | filter smallcase in a range of minimum investment amount | minInvest=5000-8000& |
risk | filter smallcase by their volatilty (can pass more than one volatility) | - risk=low& - risk=medium& - risk=high& |
recentlyLaunched | whether to display recently launched smallcase (< 1 year since launch) | recentlyLaunched=true& |
Params for sorting | description | example |
---|---|---|
sortOrder | sort in ascending / descending | - sortOrder=1& - sortOrder=-1& |
sortBy | sort by popularity | - sortBy=popularity& - sortBy=minInvestAmount& - sortBy=rebalanceDate& - sortBy=monthlyReturns& - sortBy=halfyearlyReturns& - sortBy=oneYearCagr& - sortBy=threeYearCagr& - sortBy=fiveYearCagr& |
Updated over 2 years ago